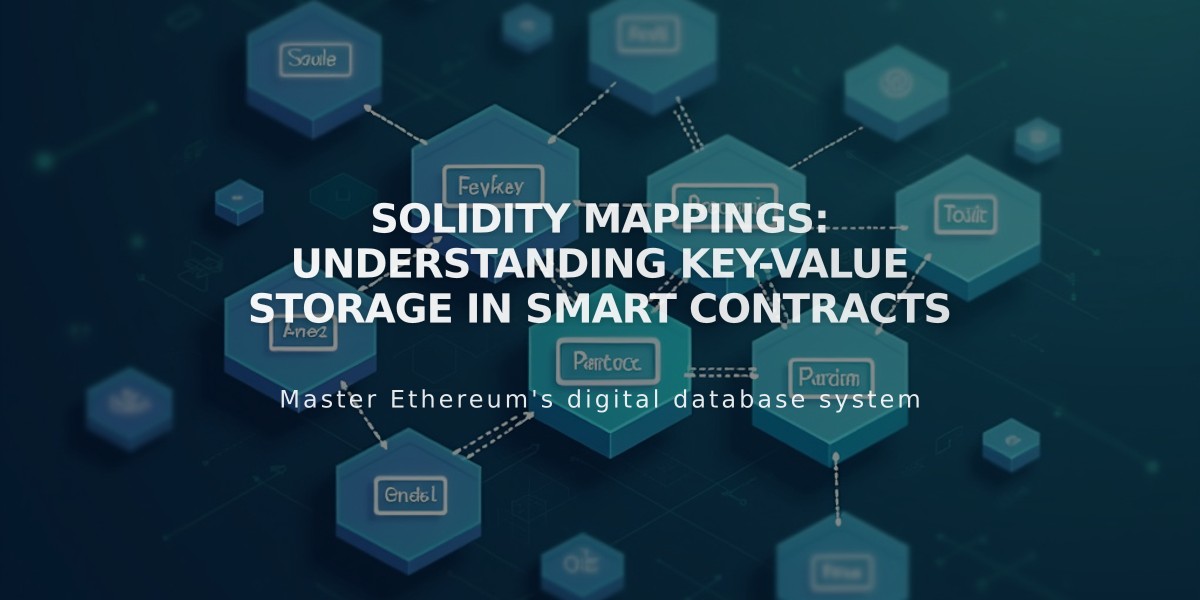
Solidity Mappings: Understanding Key-Value Storage in Smart Contracts
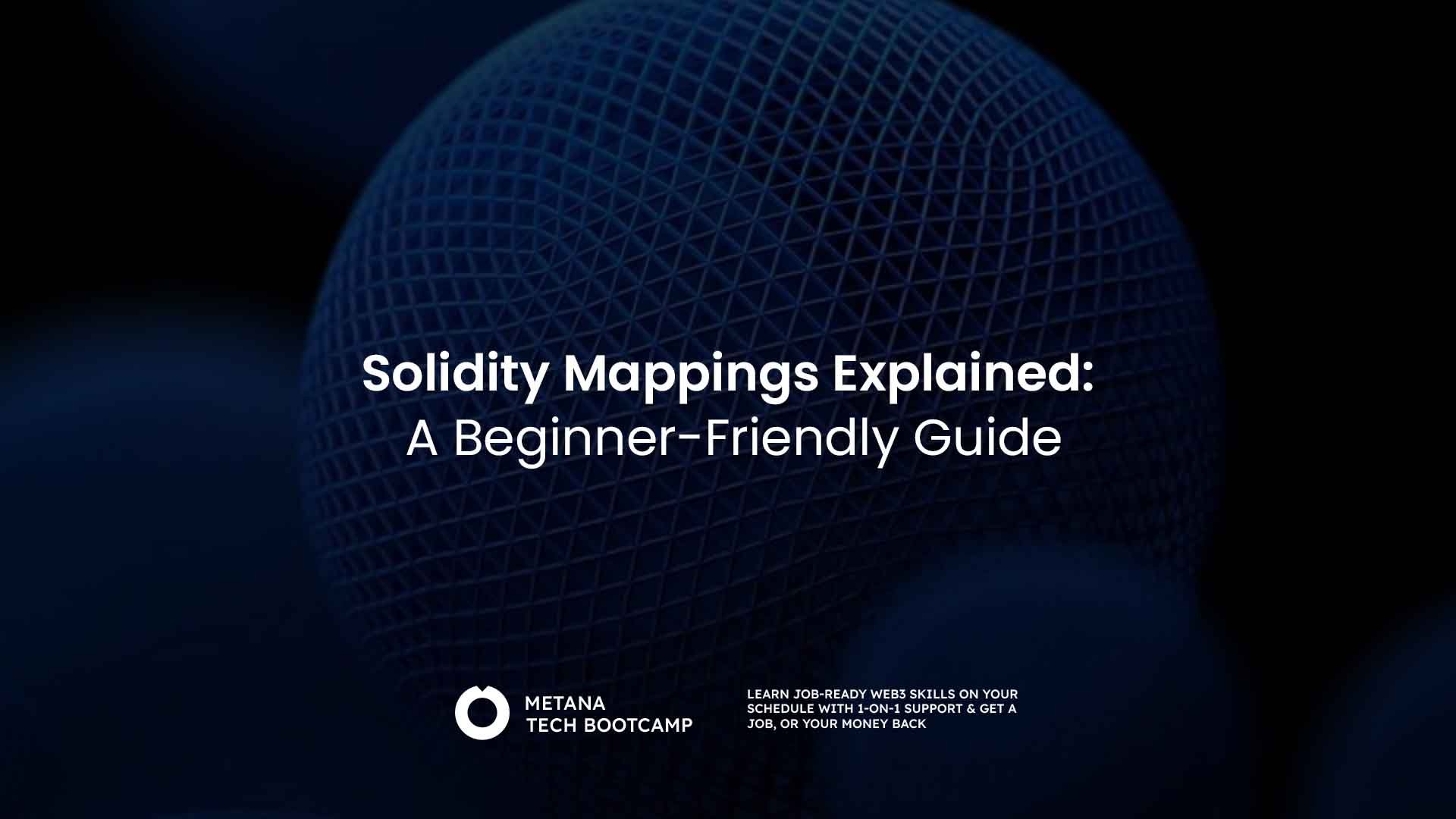
image
Mappings in Solidity are fundamental data structures that work like dictionaries or hash tables, allowing you to store and retrieve values using unique keys. They provide efficient data access and are commonly used in smart contracts.
A mapping is declared using the syntax:
mapping(KeyType => ValueType) visibility variableName
Key features of mappings:
- Lightning-fast lookups (O(1) complexity)
- Automatic initialization of values
- No size limitations
- Memory efficient storage
- Cannot be iterated over directly
Example of a basic mapping:
mapping(address => uint) public balances;
Common use cases:
- Token balances
- User permissions
- Status tracking
- Ownership records
- Price lookups
Best practices:
- Always use appropriate key types
- Initialize values explicitly
- Consider using nested mappings for complex data
- Implement getter functions for better access control
- Document mapping purposes clearly
Limitations:
- No built-in way to get all keys
- Cannot be used as function parameters or returns
- Keys are not stored and cannot be retrieved
- No way to check if a key exists
- Cannot be directly copied or compared
Using mappings efficiently can significantly improve your smart contract's performance and gas costs while maintaining data integrity and accessibility.
Related Articles
AlgoKit 3.0 Launches with TypeScript Support and Advanced Developer Tools for Algorand
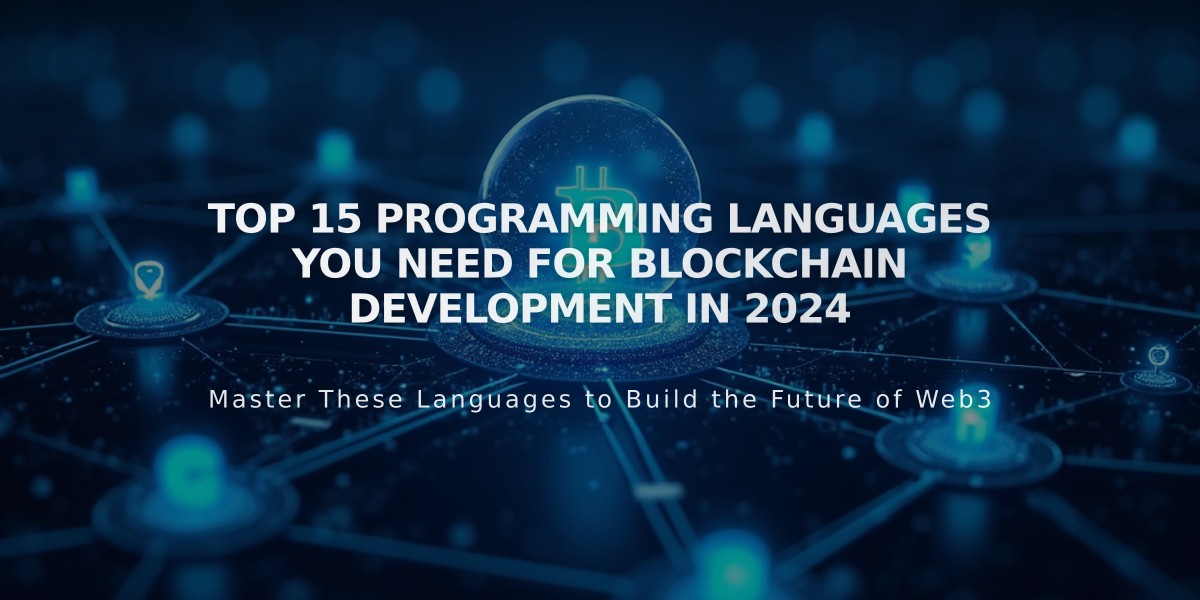