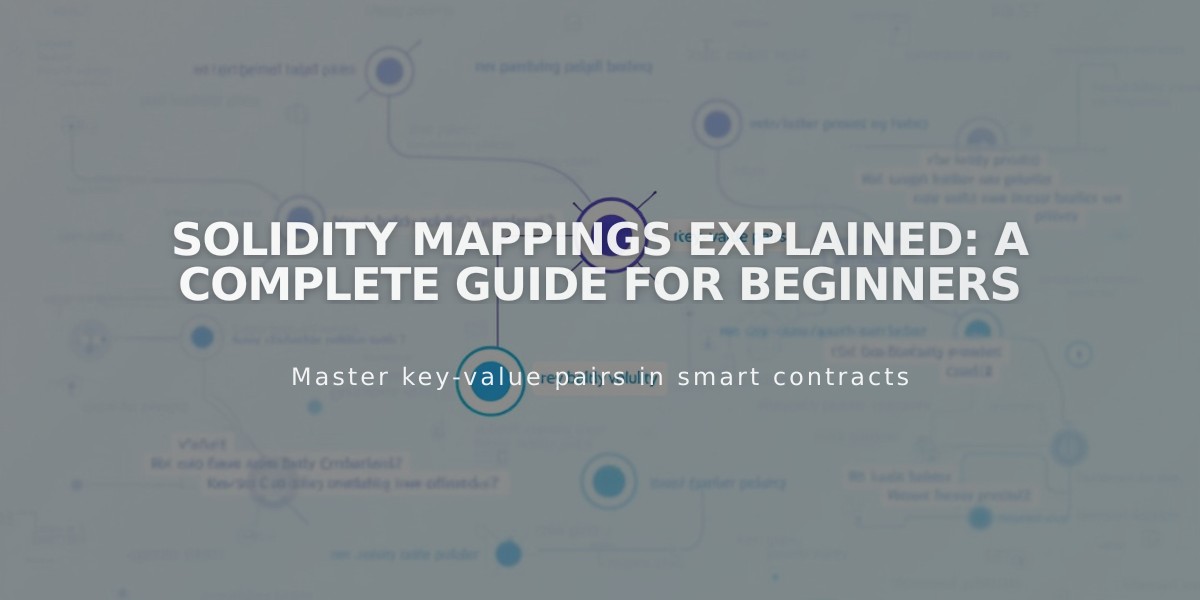
Solidity Mappings Explained: A Complete Guide for Beginners
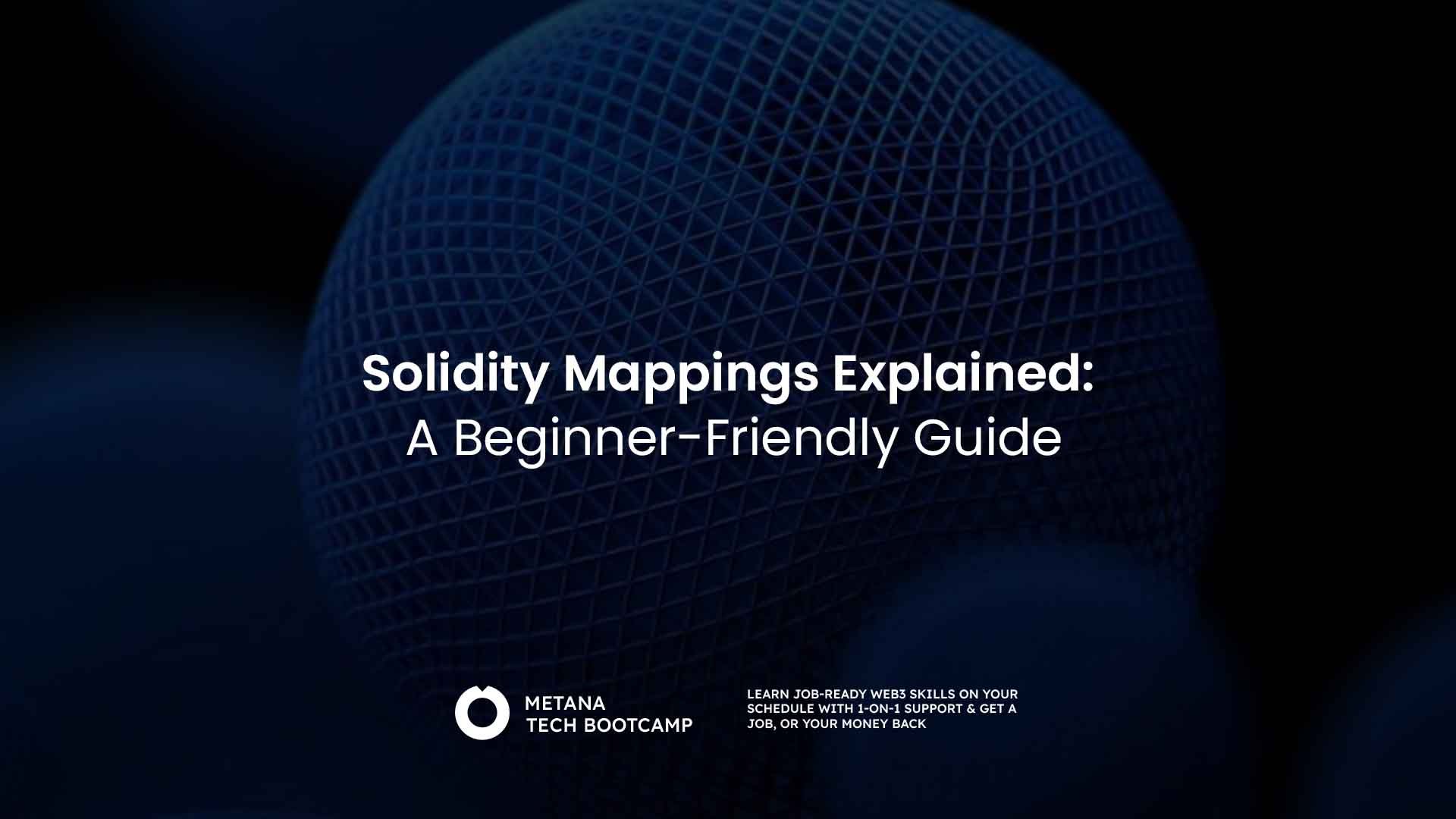
image
Mappings in Solidity are hash tables that store key-value pairs, similar to dictionaries in Python or objects in JavaScript. They provide instant data access and are memory-efficient, making them perfect for storing user balances, token ownership, and access controls in smart contracts.
Basic mapping syntax:
mapping(KeyType => ValueType) variableName;
Key features of mappings:
- Lightning-fast lookups (O(1) complexity)
- Auto-initialization of values
- Memory efficiency
- No built-in iteration support
- Keys can't be enumerated
Common use cases:
// Token balances mapping(address => uint256) public balances; // User permissions mapping(address => bool) public isAdmin; // Nested mappings for complex data mapping(address => mapping(uint256 => bool)) public tokenApprovals;
Best practices:
- Always initialize mappings as storage variables
- Use appropriate key types (address, uint, bytes32)
- Consider adding helper functions for data access
- Implement external iteration methods if needed
- Validate inputs before updating mapping values
Limitations:
- Can't get mapping length
- No direct key iteration
- Keys aren't stored (can't get list of keys)
- Values are always initialized
Example implementation:
contract TokenContract { mapping(address => uint256) public balances; function transfer(address to, uint256 amount) public { require(balances[msg.sender] >= amount, "Insufficient balance"); balances[msg.sender] -= amount; balances[to] += amount; } }
Mappings are fundamental to Solidity development, providing efficient data storage and retrieval for decentralized applications. While they lack some features like iteration, their speed and simplicity make them invaluable for most smart contract use cases.
Related Articles
AlgoKit 3.0 Launches with TypeScript Support and Advanced Developer Tools for Algorand
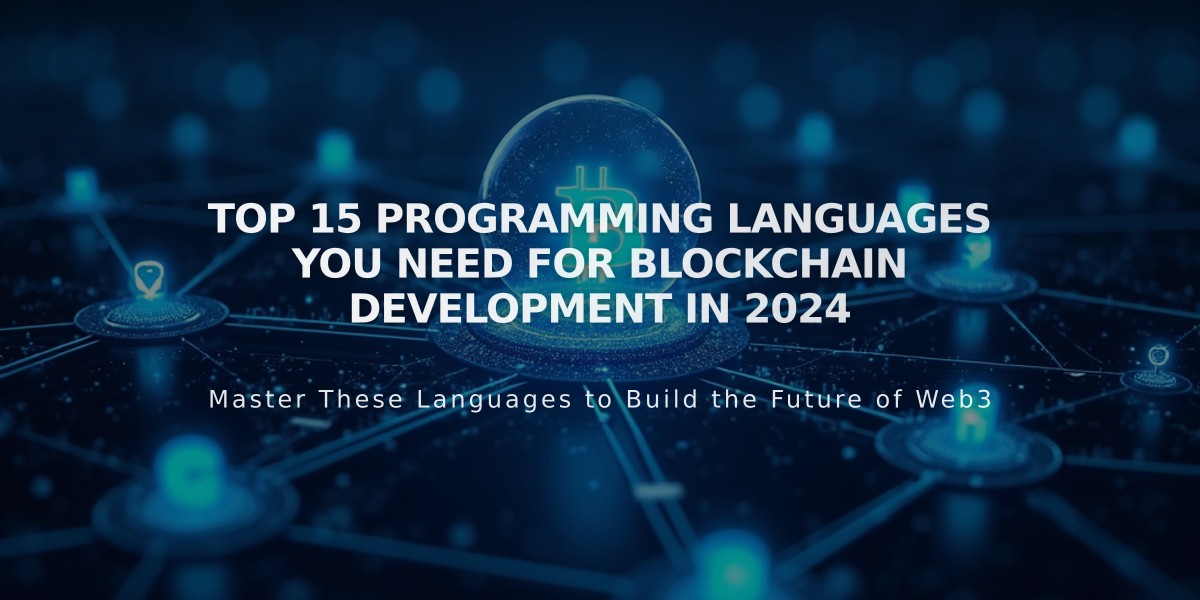