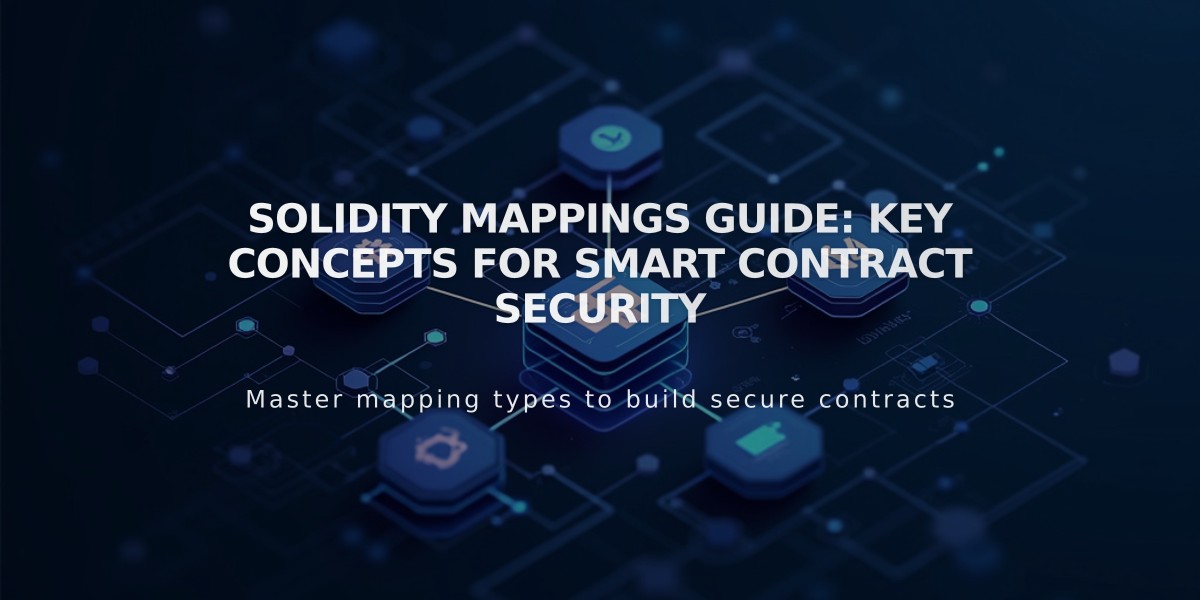
Solidity Mappings Guide: Key Concepts for Smart Contract Security
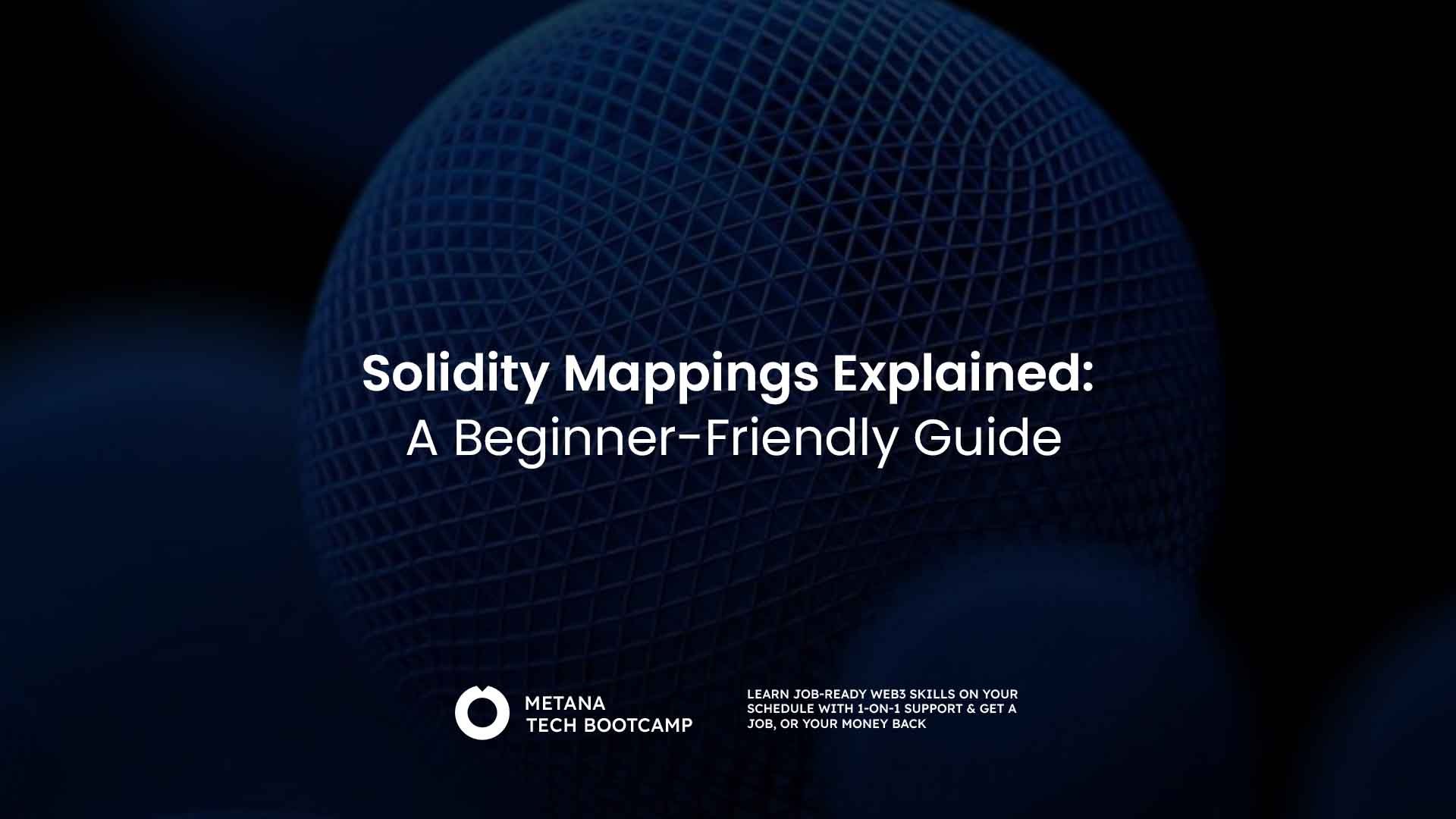
image
Mappings in Solidity serve as key-value pair data structures that enable efficient data storage and retrieval in smart contracts. They function similarly to hash tables or dictionaries in other programming languages.
A mapping uses the syntax
mapping(KeyType => ValueType)where KeyType can be any built-in value type, bytes, string, or contract, while ValueType can be any type including another mapping or array.
Basic example of a mapping:
mapping(address => uint256) public balances;
Key features of mappings:
- Fast lookups using unique keys
- Automatic initialization of values to their default state
- No way to iterate through all entries
- Cannot determine size/length
- Keys are not stored, only their keccak256 hashes
Common use cases:
- Token balances
- User permissions and roles
- Game state storage
- Contract ownership tracking
- Price lookups
Example implementation:
contract ExampleMapping { mapping(address => uint) public userBalances; function deposit() public payable { userBalances[msg.sender] += msg.value; } function getBalance() public view returns (uint) { return userBalances[msg.sender]; } }
Nested mappings are also possible for more complex data structures:
mapping(address => mapping(uint => bool)) public nestedExample;
Best practices:
- Always initialize mappings in the contract's state variables
- Use appropriate key types for your use case
- Consider gas costs when choosing value types
- Include proper access controls
- Be mindful of potential key collisions
Mappings are immutable and cannot be deleted entirely, though individual key-value pairs can be reset to their default values. They provide a gas-efficient way to store and access data in smart contracts, making them essential for many blockchain applications.
Related Articles
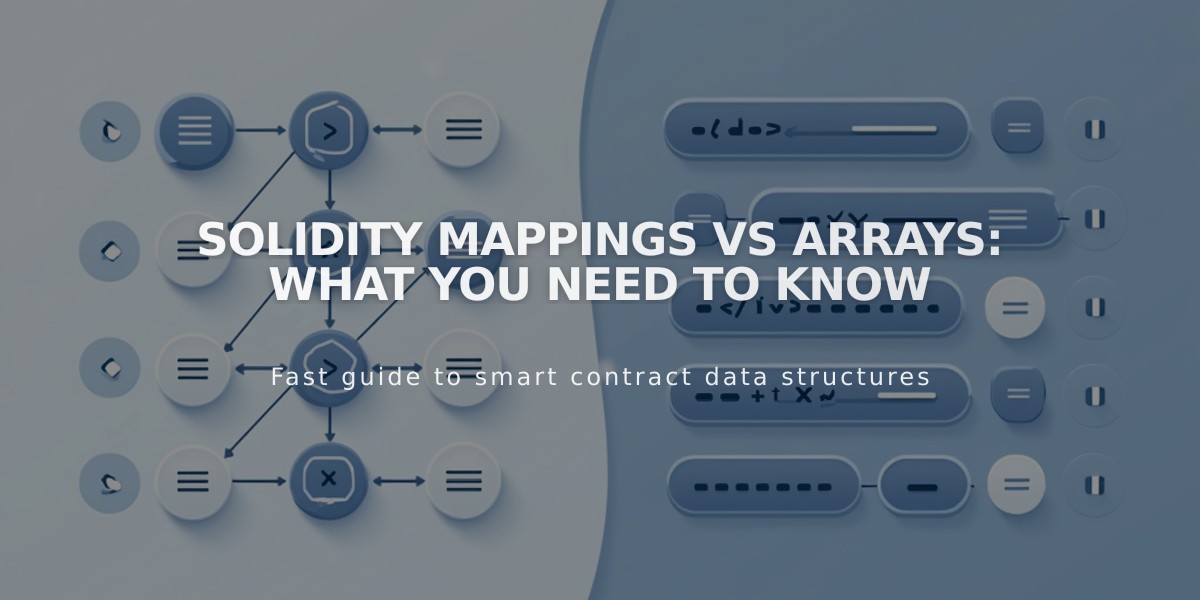
Solidity Mappings vs Arrays: What You Need to Know
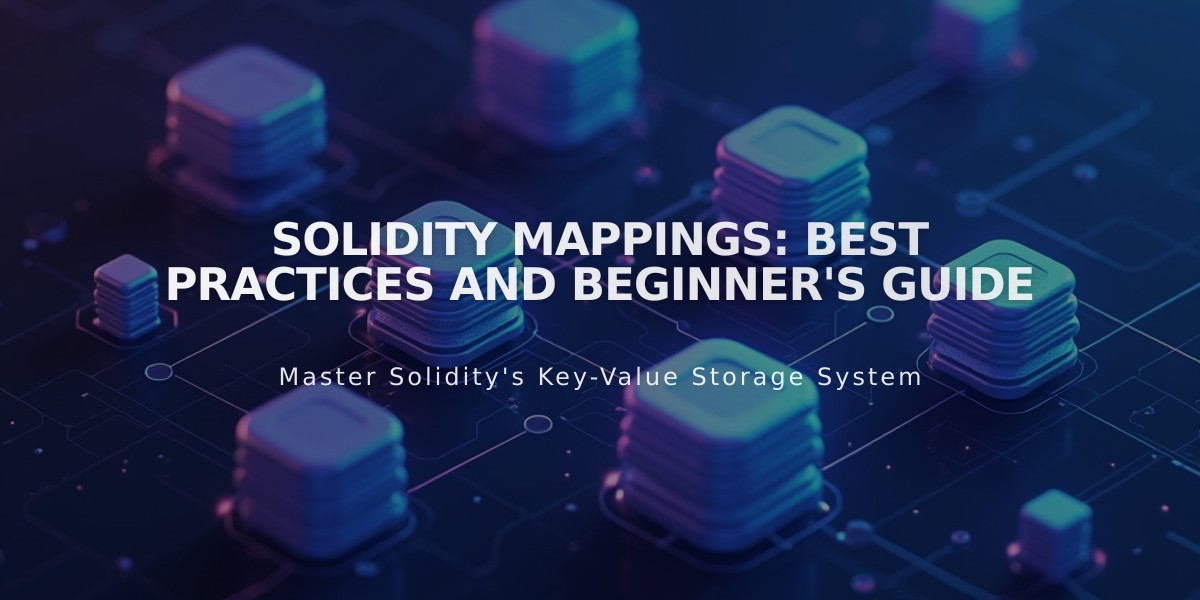