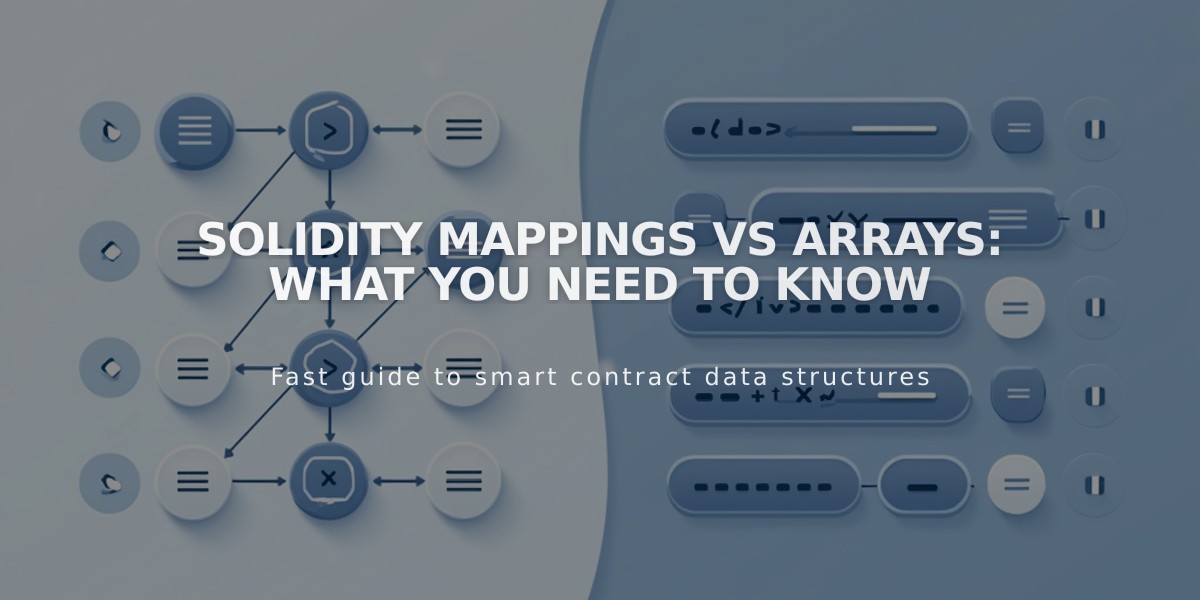
Solidity Mappings vs Arrays: What You Need to Know
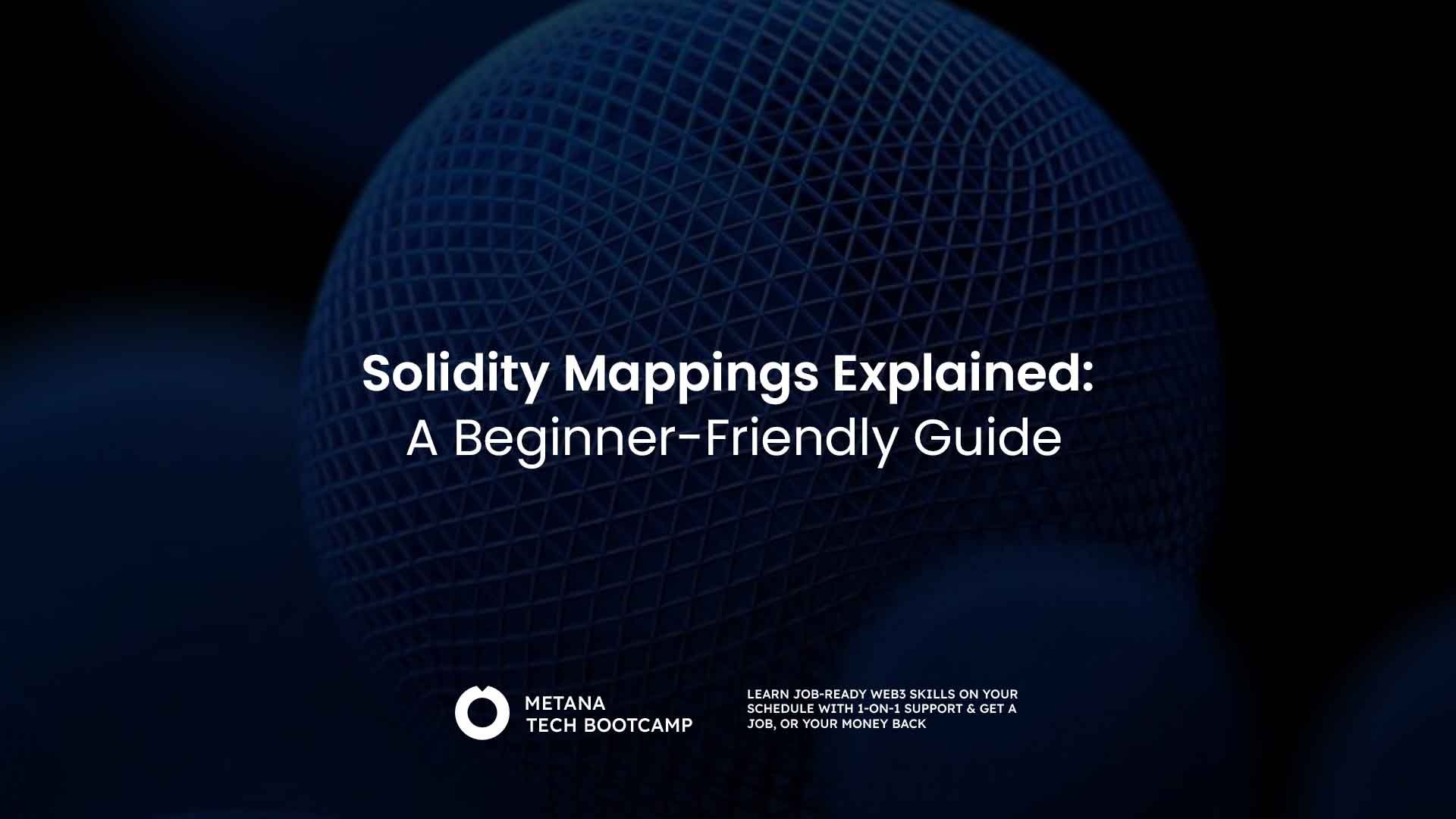
image
Mappings in Solidity act as hash tables that store key-value pairs, similar to dictionaries in Python or objects in JavaScript. They provide instant value lookup using unique keys and are gas-efficient for smart contract storage.
Basic mapping syntax:
mapping(keyType => valueType) visibility variableName;
Example of a token balance mapping:
mapping(address => uint256) public balances;
Key features of Solidity mappings:
- Zero-value initialization: All possible keys exist by default with zero/null values
- No size tracking: Mappings don't store length or key lists
- Key immutability: Keys must be value types, not reference types
- Direct key access: No iteration through keys possible
- Gas efficiency: Optimized for single-value lookups
Common use cases:
- Token balances
- User permissions
- Game state storage
- Voting systems
- Contract ownership records
Best practices:
- Always validate input data before storing
- Use appropriate key and value types
- Consider gas costs for complex operations
- Document mapping purposes clearly
- Implement getter functions for better access control
Note: Unlike arrays, mappings cannot be iterated over directly. If you need to track or iterate through stored values, maintain a separate array of keys.