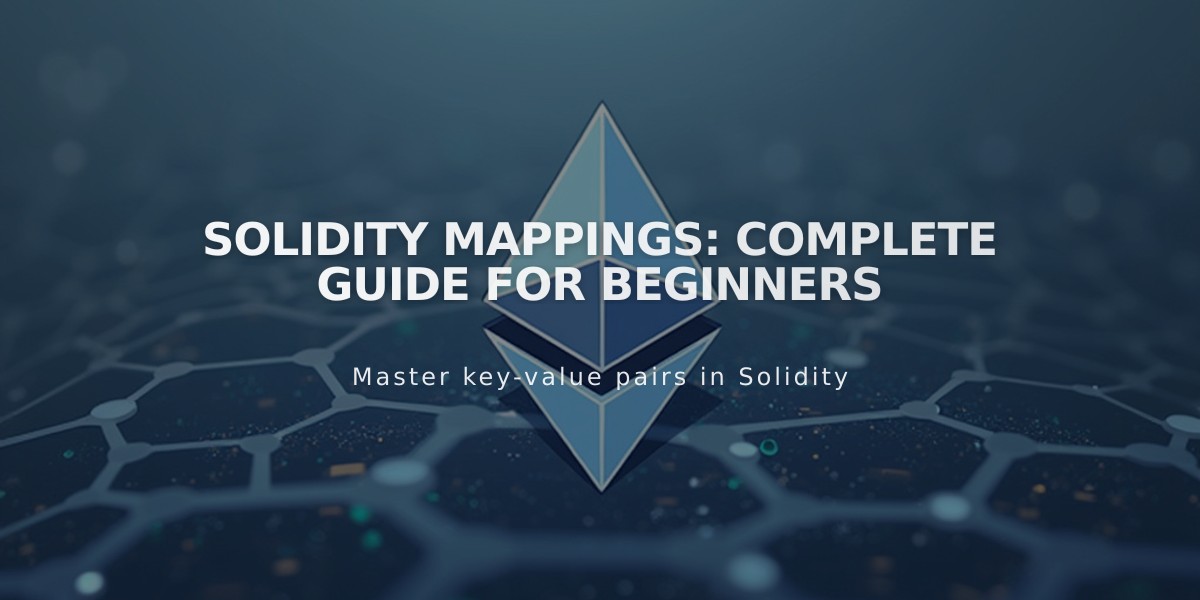
Solidity Mappings: Complete Guide for Beginners
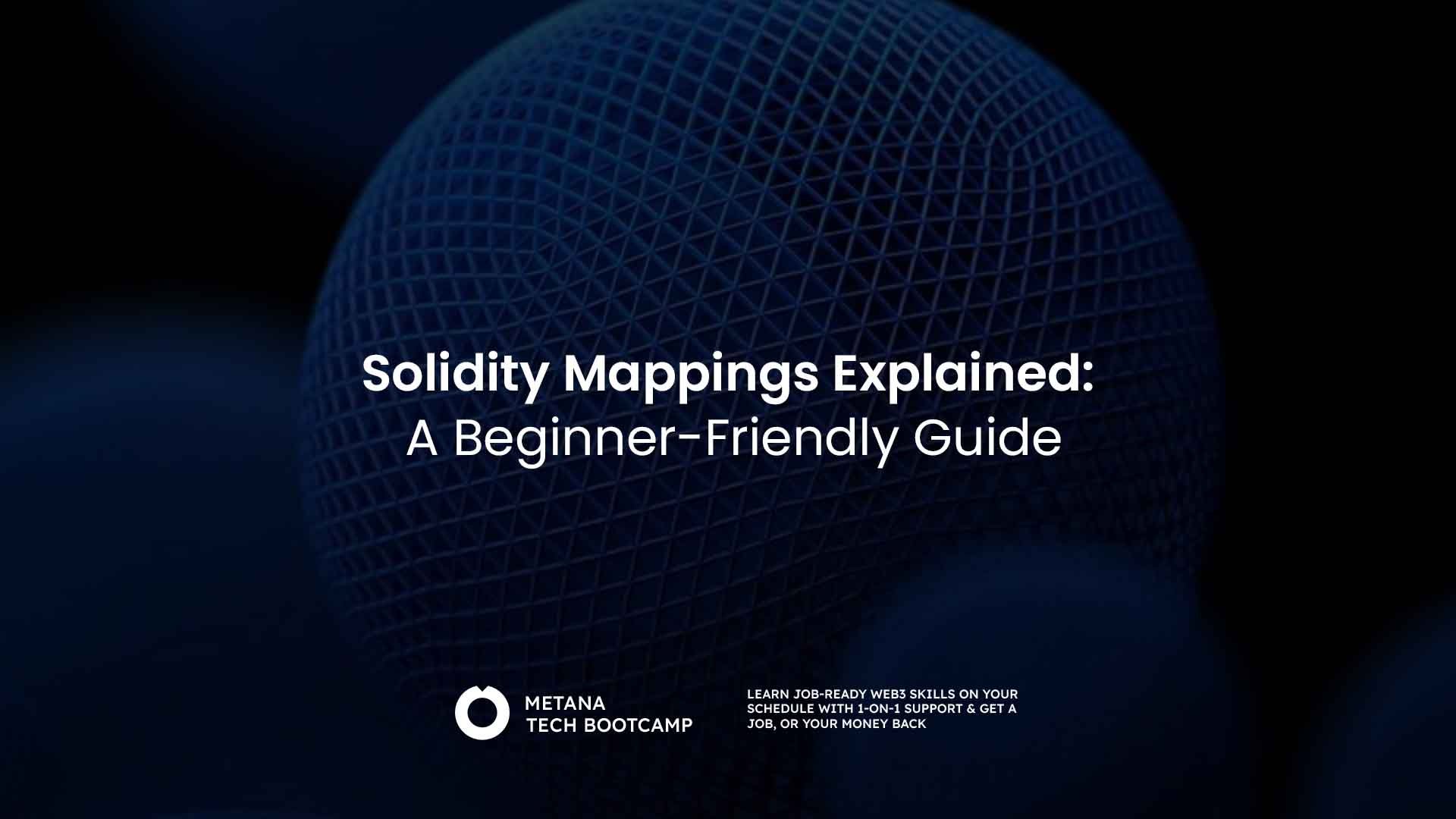
image
Mappings are fundamental data structures in Solidity that function as hash tables, storing key-value pairs with instant data retrieval. They're essential for building efficient smart contracts on Ethereum.
A mapping is declared using the syntax
mapping(KeyType => ValueType) VariableName. For example:
mapping(address => uint) public balances;
Key features of Solidity mappings:
- Instant value lookup using keys
- Cannot be iterated over directly
- All possible keys exist by default (initialized to zero/empty)
- Key-value pairs are not actually stored in memory
Common use cases:
- Token balances tracking
- User permissions management
- State tracking for addresses
- Relationship mapping between different data types
Example implementation for a basic token contract:
mapping(address => uint256) public balances; function transfer(address recipient, uint256 amount) public { require(balances[msg.sender] >= amount); balances[msg.sender] -= amount; balances[recipient] += amount; }
Best practices:
- Always initialize mappings in the contract's constructor
- Use appropriate key types (address, uint, bytes32 are common)
- Include proper access controls
- Consider using nested mappings for complex data structures
Important limitations:
- Cannot get a list of all keys
- No built-in way to determine size
- Keys are not stored in the blockchain
- Values can only be accessed if you know the key
Mappings are memory-efficient since they only store non-zero values, making them perfect for sparse data sets like token balances or user permissions in decentralized applications.
Related Articles
AlgoKit 3.0 Launches with TypeScript Support and Advanced Developer Tools for Algorand
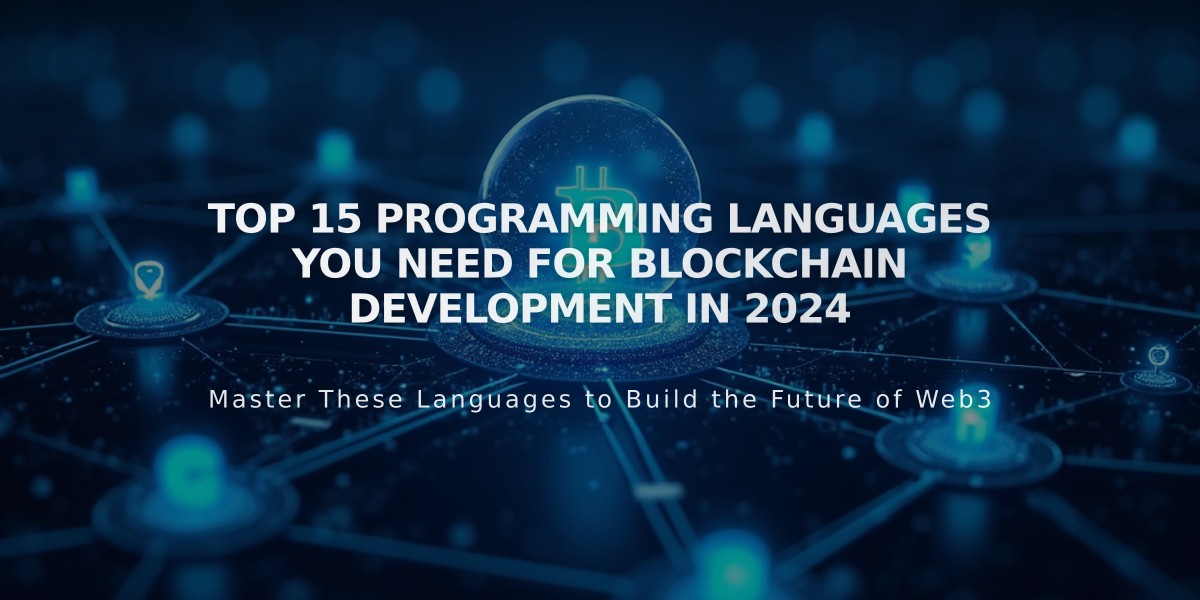