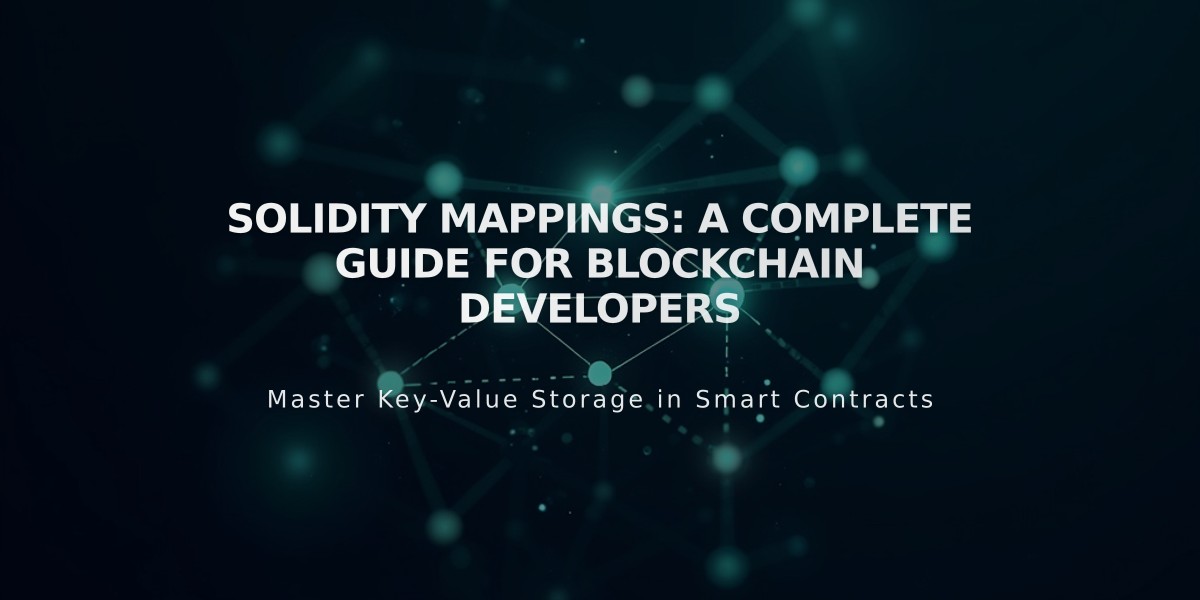
Solidity Mappings: A Complete Guide for Blockchain Developers
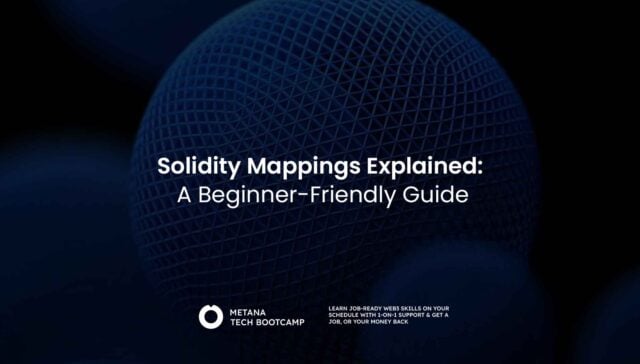
image
Mappings in Solidity serve as hash tables that store key-value pairs, similar to dictionaries in Python or objects in JavaScript. They enable efficient data storage and retrieval in smart contracts.
A mapping is declared using the syntax:
mapping(KeyType => ValueType) VariableName. The KeyType can be any built-in value type, while ValueType can be any type, including another mapping or array.
mapping(address => uint) public balances; mapping(uint => string) public names; mapping(address => mapping(uint => bool)) public nestedMapping;
Key characteristics of mappings:
- All possible keys exist by default
- Keys are not stored in the mapping
- Values are initialized to their default type value
- Cannot iterate over mappings directly
- Cannot get the size/length of a mapping
Example implementation:
contract MappingExample { mapping(address => uint) public userBalances; function deposit() public payable { userBalances[msg.sender] += msg.value; } function getBalance() public view returns (uint) { return userBalances[msg.sender]; } }
Best practices for using mappings:
- Use when you need key-value pair storage
- Choose appropriate key types for efficient lookups
- Consider gas costs when working with nested mappings
- Implement additional tracking if iteration is needed
- Always validate inputs when modifying mapping values
Mappings are fundamental for building complex smart contracts, especially for tracking user balances, permissions, and game states. Understanding their limitations and proper usage is essential for efficient smart contract development.
Related Articles
AlgoKit 3.0 Launches with TypeScript Support and Advanced Developer Tools for Algorand
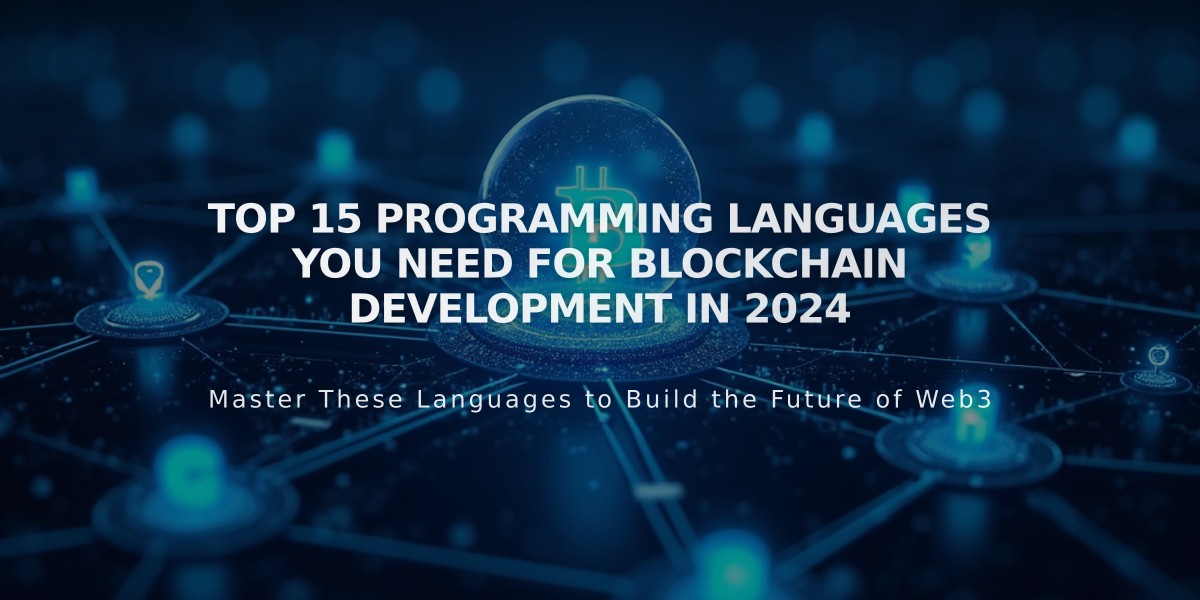